Rejuvenating/Refactoring Legacy Enterprise Applications
The following blog appeared on my previous blog site (August 2015) and I thought I would repost on this site
I have just finished a 7 month assignment, which originally started as a short 4 week consultancy assignment for a company ladened with ‘Green Screens’. Working there reminded me of the early 80’s when I worked at a British Bank writing queries on the IBM 3090 mainframes & GEAC Mini using both Easytrieve and KARL, to produce similar code logic to validate the Banks Lease migration data.
Earlier this year I produced a ‘briefing note’, in which I suggested a technical direction for the AS400 platform and how the Report Program Generator (RPG) could / should be transposed to the updated iSeries JAVA platform – today’s blog is a slight re-use of extracts from the original note with the aim of sharing some thoughts on giving a legacy AS400 system a new lease of life through the extension and refactoring of core software components.
My client continues to add to its technical debt by constructing, extending and enhancing processes and procedures around its AS400 (rebranded as the IBM iSeries) resulting in a platform that lacks economic sustainability in the long run due to availability of support and further enhancements, especially resulting from the lack of good RPG programmers.
RPG falls into the category of a Procedural Programming Language and comprises of one or more units or modules following strict syntax rules of calling predefined functions from the code library; each module is composed of one or more procedures or functions, routines, subroutines, or methods
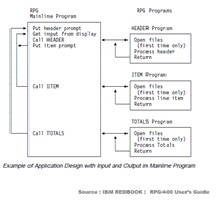
The iSeries is a solid execution platform and RPG has stood the test of time, maturing to provide a stable development / operational environment to deliver industrial strength Business Systems. However the iSeries has evolved to run alternative Operating Systems such as Linux and execute modern Object Orientated Languages such as Java.
If you still have RPG/AS400 supporting some of your company’s core processes it may be time to consider or ‘dip your toes in the water’ by refactoring small non critical applications. My recommendation would be avoid applications which are heavily reliant on large volumes of business rules, logic and maybe start small with recreating RPG reports, applying a move at each layer of the legacy Architectural stack.
![]() |
RPG - Java Tiers |
Reporting is easiest as you retrieve, perform some calculations, structure and render the information which would only require a small subset of functionality exploiting existing Java Data manipulation capabilities on the iSeries or write a Java app that calls the RPG via a API Call (Discussed below).
A desired outcome for refactoring RPG Code to java is to replicate the modules without changing external behaviour, thus functionally invisible to the users other than update of the presentation tier (Green screen to Browser/GUI) for end user apps.
Re-writing of the code in Java will eventually reduce future development time and cost through reusing code effort i.e. once an object class is created in Java it will be used/extended for other applications resulting in easing the effort associated with debugging / testing code as classes can be tested independently and then reused.
IBM has made life a lot easier and has provided an easy to use library for communicating with the iSeries from Java- See The IBM Toolbox for Java , a library of Java classes that provide easy access to IBM iSeries data and resources. JT Open is the open source version of Toolbox for Java.
Above I am discussing recreating the business/app logic and not normalisation or creation of any new data structures and the Database Architecture (Tables, Views, and Stored Procedures etc.) is ignored during the proof of concept as revisiting existing data schemas is not a small task especially as other applications often share tables.
There are numerous patterns which can be adopted when considering the refactoring of legacy code and the depiction below illustrates three standard patterns.
Pattern | Env | Comments | Useful URLS |
1 | Desktop – thick client | Maintains core business logic , however uses the RPG API available to the Java developer The Client would make http Service Calls to the iSeries | Building a REST service with integrated web services server for IBM i: Part 2 |
2 | Mid-Tier deploymenti.e. The Java Code is developed / deployed on the iSeries | The iSeries acts as the client system and execute java code locally utilising all local system resources. No RPG code is executed (except reporting – see note below) and this is a complete re-write of the RPG modules The IBM Toolbox for Java ( Java Classes under /QIBM/ProdData/OS400/jt400/) ) must be available to the IBM I Host Servers
| http://www-01.ibm.com/support/knowledgecenter/ssw_ibm_i_61/rzaha/whatitis.htm |
3 | JEE App Server proxy | App Server acting as a proxy between the iSeries and the Client with a gradual move of business logic to the JEE instance (which could be on the iSeries) |
Pattern 1 – Direct API Calls – reusing RPG Logic Components
A thick client on the desktop invokes RPG Code via API Calls This is shown in the diagram below (for illustration only) where the NewApp() java component talks direct to iSeries to invoke the RPG modules via the API mechanism
The NewApp() component would ‘mimic’ the original RPG Program (menu items) and execute the relevant RPG modules monitoring for exceptions (errors).
The code sample (below) is slightly modified from the source available on IBMs Developer Works Site however highlights the mechanism in operation, for more Information on the iSeries RPG API refer to the IBM Redbook ;
Sample Code : Program Name, Library Name, PGM Location to be defined
/* Start */
import java.util.Date;
import com.ibm.as400.access.AS400;
import com.ibm.as400.access.AS400Message;
import com.ibm.as400.access.ProgramCall;
import com.ibm.as400.access.ProgramParameter;
/* Sample program to test the RPG call from Java. Modified from Original source at IBM Developer Works */
public class AS400Test {
public static void main(String[] args) {
String server=”xxxxxxxxxx”; /* replace with your test server */
String user = “AS400USER”; /* USER Account on AS400 */
String pass = “AS400PWRD”;
String input = “SEAT”;
String fullProgramName = “/QSYS.LIB/SEAT.LIB/SEATPGM.PGM”;
/* replace with the path for Programme Libraries */
AS400 as400 = null;
try {
// Create an AS400 object
as400 = new AS400(server, user, pass);
// Create a parameter list
// The list must have both the input and output parameters
ProgramParameter[] parmList = new ProgramParameter[2];
// Convert the Strings to IBM format
byte[] inData = input.getBytes(“IBM285”);
// Create the input parameter
parmList[0] = new ProgramParameter(inData);
// Create the output parameter
parmList[1] = new ProgramParameter(5);
// Create a program object specifying the name of the program and the parameter list.
ProgramCall pgm = new ProgramCall(as400);
pgm.setProgram(fullProgramName, parmList);
// Run the program.
if (!pgm.run()) {
// If the AS/400 cannot run the program, look at the message list to find out why it didn’t run.
AS400Message[] messageList = pgm.getMessageList();
for (AS400Message message : messageList) {
System.out.println(message.getID() + ” – ” + message.getText());
}
}
else {
// Else the program ran. Process the second parameter, which contains the returned data.
byte[] data = parmList[1].getOutputData();
String lastName = new String(data, “IBM285”).trim();
System.out.println(“Output is ” + lastName);
}
} catch (Exception e) {
e.printStackTrace();
}finally{
try{
// disconnect
as400.disconnectAllServices();
}catch(Exception e){}
}
System.exit(0);
}
}
/* End */
Note : The compiler will ignore everything from // to the end of the line
Reporting
Reporting for NewApp_J() is a Special Use Case – as it would not be prudent to re-write the reporting modules. However as new reporting modules are required these can be re-developed in Java.
The reporting module acts on static data and thus requires no change to the RPG (check this)
Pattern 2: Direct Execution of Java Code on the AS400 (iSeries)
Pattern 2 is similar to Pattern 1, however, the code is executed on the AS400 (iSeries) itself where the containers for the code and execution are hosted on the AS400 thus mitigating client side risk.
The following Sequence is similar to the one depicted above, but highlights the typical local flow between a legacy RPG system and the Java System. However introduce Interactive Structured Query Language (ISQL), a product, which sits on top of SQL allowing you to leverage SQL commands interactively (worth a look at if you proceed to do a proof of concept).
Each interaction should be re-engineered as per the RPG Legacy App menu, so analysis of existing screens and procedures will supply the input for the design of the new java app.
Pattern 3 – Introduction of a mid-tier JEE platform
A common pattern and worthy of a whole blog in itself – However IBM have written enough Redbooks that you can review.
If you have time – have a look at IBM – Rational Host Access Transformation Services(HATS) a single, low risk solution that provides the tools and runtime support that you need to quickly and easily extend your 3270 and 5250 green screen.
In Summary
There are many ways in which you can rejuvenate, refactor and re-write your legacy applications. If your system is legacy IBM, then you’re in luck as the guys at IBM have done an excellent job in providing material and samples which allow you to get started in extending the life of your systems.
I was asked to explore how a Business that orchestrates all of its processes around a single central Enterprise System could move forward – one of many recommendations was to explore the new capabilities offered by the iSeries and java was the strong contender.
clearly one size does not fit all and the purpose of the above is just to provide ‘food for thought.
I hope you found the above and the various URLs useful.
Comments
Post a Comment